How To Pass Command Line Arguments In Dev C++
If no arguments are supplied, argc will be one, and if you pass one argument then argc is set at 2. You pass all the command line arguments separated by a space, but if argument itself has a space then you can pass such arguments by putting them inside double quotes ' or single quotes '. Nov 10, 2019 However, we can also pass arguments to the main function of C which are known as Command Line Arguments. Command line arguments are given after the name of the program during the execution of the program in a command-line shell. In order to pass command line arguments, the main function is passed with two arguments. Aug 07, 2009 Adding the ability to parse command-line parameters to a program is very easy. Every C and C program has a main function. It is always at least 1, because the first string in argv ( argv 0) is the command used to invoke the program. Argv contains the actual command-line arguments as. The following are the steps to execute a program with Command Line Argument inside Turbo C/C Compiler: 1. Open Turbo C. Open Turbo C. If you don't have, you can download Turbo C from here. Write a program. Compile and Run your program. Open DOS Shell. Change directory. Command Line Argument in C. Command line argument is a parameter supplied to the program when it is invoked. Command line argument is an important concept in C programming. It is mostly used when you need to control your program from outside. Command line arguments are passed to the main method. Syntax: int main(int argc, char.argv). Apr 25, 2008 Hey, Scripting Guy! I am trying to write a script that requests multiple pieces of information from a user; I then want to call a batch file, using each piece of information as a separate command-line argument. Unfortunately, though, I can’t get this to work correctly.
-->All C++ programs must have a main
function. If you try to compile a C++ .exe project without a main function, the compiler will raise an error. (Dynamic-link libraries and static libraries don't have a main
function.) The main
function is where your source code begins execution, but before a program enters the main
function, all static class members without explicit initializers are set to zero. In Microsoft C++, global static objects are also initialized before entry to main
. Several restrictions apply to the main
function that do not apply to any other C++ functions. The main
function:
- Cannot be overloaded (see Function Overloading).
- Cannot be declared as inline.
- Cannot be declared as static.
- Cannot have its address taken.
- Cannot be called.
The main function doesn't have a declaration, because it's built into the language. If it did, the declaration syntax for main
would look like this:
Microsoft Specific
If your source files use Unicode wide characters, you can use wmain
, which is the wide-character version of main
. The declaration syntax for wmain
is as follows:
You can also use _tmain
, which is defined in tchar.h. _tmain
resolves to main
unless _UNICODE is defined. In that case, _tmain
resolves to wmain
.
If no return value is specified, the compiler supplies a return value of zero. Alternatively, the main
and wmain
functions can be declared as returning void (no return value). If you declare main
or wmain
as returning void, you cannot return an exit code to the parent process or operating system by using a return statement. To return an exit code when main
or wmain
is declared as void, you must use the exit function.
END Microsoft Specific
Command line arguments
The arguments for main
or wmain
allow convenient command-line parsing of arguments and, optionally, access to environment variables. The types for argc
and argv
are defined by the language. The names argc
, argv
, and envp
are traditional, but you can name them whatever you like.
The argument definitions are as follows:
argc
An integer that contains the count of arguments that follow in argv. The argc parameter is always greater than or equal to 1.
Our team performs checks each time a new file is uploaded and periodically reviews files to confirm or update their status. At the end of the workshop, if you have pulled it off, then you can be sure of some happy customers and a pat on the back from the head chef. Free download game cooking academy 2 for pc full version 1 8. In Softonic we scan all the files hosted on our platform to assess and avoid any potential harm for your device. Each procedure is a game in its own right, and you will need to prepare for a fast-paced experience as you prepare each ingredient at a time.
argv
An array of null-terminated strings representing command-line arguments entered by the user of the program. By convention, argv[0]
is the command with which the program is invoked, argv[1]
is the first command-line argument, and so on, until argv[argc]
, which is always NULL. See Customizing Command Line Processing for information on suppressing command-line processing.
The first command-line argument is always argv[1]
and the last one is argv[argc - 1]
.
Note
By convention, argv[0]
is the command with which the program is invoked. However, it is possible to spawn a process using CreateProcess and if you use both the first and second arguments (lpApplicationName and lpCommandLine), argv[0]
may not be the executable name; use GetModuleFileName to retrieve the executable name, and its fully-qualified path.
Microsoft Specific
envp
The envp array, which is a common extension in many UNIX systems, is used in Microsoft C++. It is an array of strings representing the variables set in the user's environment. This array is terminated by a NULL entry. It can be declared as an array of pointers to char (char *envp[]
) or as a pointer to pointers to char (char **envp
). If your program uses wmain
instead of main
, use the wchar_t data type instead of char. The environment block passed to main
and wmain
is a 'frozen' copy of the current environment. If you subsequently change the environment via a call to putenv
or _wputenv
, the current environment (as returned by getenv
or _wgetenv
and the _environ
or _wenviron
variable) will change, but the block pointed to by envp will not change. See Customizing Command Line Processing for information on suppressing environment processing. This argument is ANSI compatible in C, but not in C++.
END Microsoft Specific
Example
The following example shows how to use the argc, argv, and envp arguments to main
:
Parsing C++ command-Line arguments
Microsoft Specific
Microsoft C/C++ startup code uses the following rules when interpreting arguments given on the operating system command line:
Arguments are delimited by white space, which is either a space or a tab.
The caret character (^) is not recognized as an escape character or delimiter. The character is handled completely by the command-line parser in the operating system before being passed to the
argv
array in the program.A string surrounded by double quotation marks ('string') is interpreted as a single argument, regardless of white space contained within. A quoted string can be embedded in an argument.
A double quotation mark preceded by a backslash (') is interpreted as a literal double quotation mark character (').
Backslashes are interpreted literally, unless they immediately precede a double quotation mark.
If an even number of backslashes is followed by a double quotation mark, one backslash is placed in the
argv
array for every pair of backslashes, and the double quotation mark is interpreted as a string delimiter.If an odd number of backslashes is followed by a double quotation mark, one backslash is placed in the
argv
array for every pair of backslashes, and the double quotation mark is 'escaped' by the remaining backslash, causing a literal double quotation mark (') to be placed inargv
.
Example
The following program demonstrates how command-line arguments are passed:
The following table shows example input and expected output, demonstrating the rules in the preceding list.
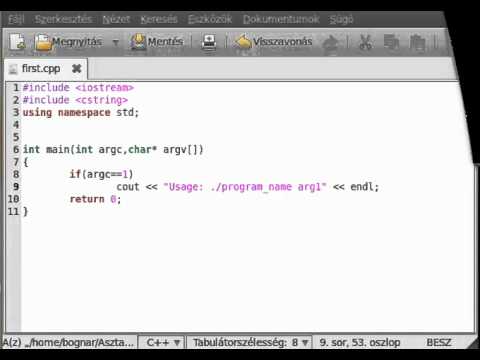
Results of parsing command lines
Command-Line Input | argv[1] | argv[2] | argv[3] |
---|---|---|---|
'abc' d e | abc | d | e |
ab d'e f'g h | ab | de fg | h |
a'b c d | a'b | c | d |
a'b c' d e | ab c | d | e |
END Microsoft Specific
Wildcard expansion
Microsoft Specific
You can use wildcards — the question mark (?) and asterisk (*) — to specify filename and path arguments on the command-line.
Command-line arguments are handled by a routine called _setargv
(or _wsetargv
in the wide-character environment), which by default does not expand wildcards into separate strings in the argv
string array. For more information on enabling wildcard expansion, refer to Expanding Wildcard Arguments.
END Microsoft Specific
Customizing C++ command-line processing
Microsoft Specific
If your program does not take command-line arguments, you can save a small amount of space by suppressing use of the library routine that performs command-line processing. This routine is called _setargv
and is described in Wildcard Expansion. To suppress its use, define a routine that does nothing in the file containing the main
function, and name it _setargv
. The call to _setargv
is then satisfied by your definition of _setargv
, and the library version is not loaded.
Similarly, if you never access the environment table through the envp
argument, you can provide your own empty routine to be used in place of _setenvp
, the environment-processing routine. Just as with the _setargv
function, _setenvp
must be declared as extern 'C'.
Your program might make calls to the spawn
or exec
family of routines in the C run-time library. If it does, you shouldn't suppress the environment-processing routine, since this routine is used to pass an environment from the parent process to the child process.
END Microsoft Specific